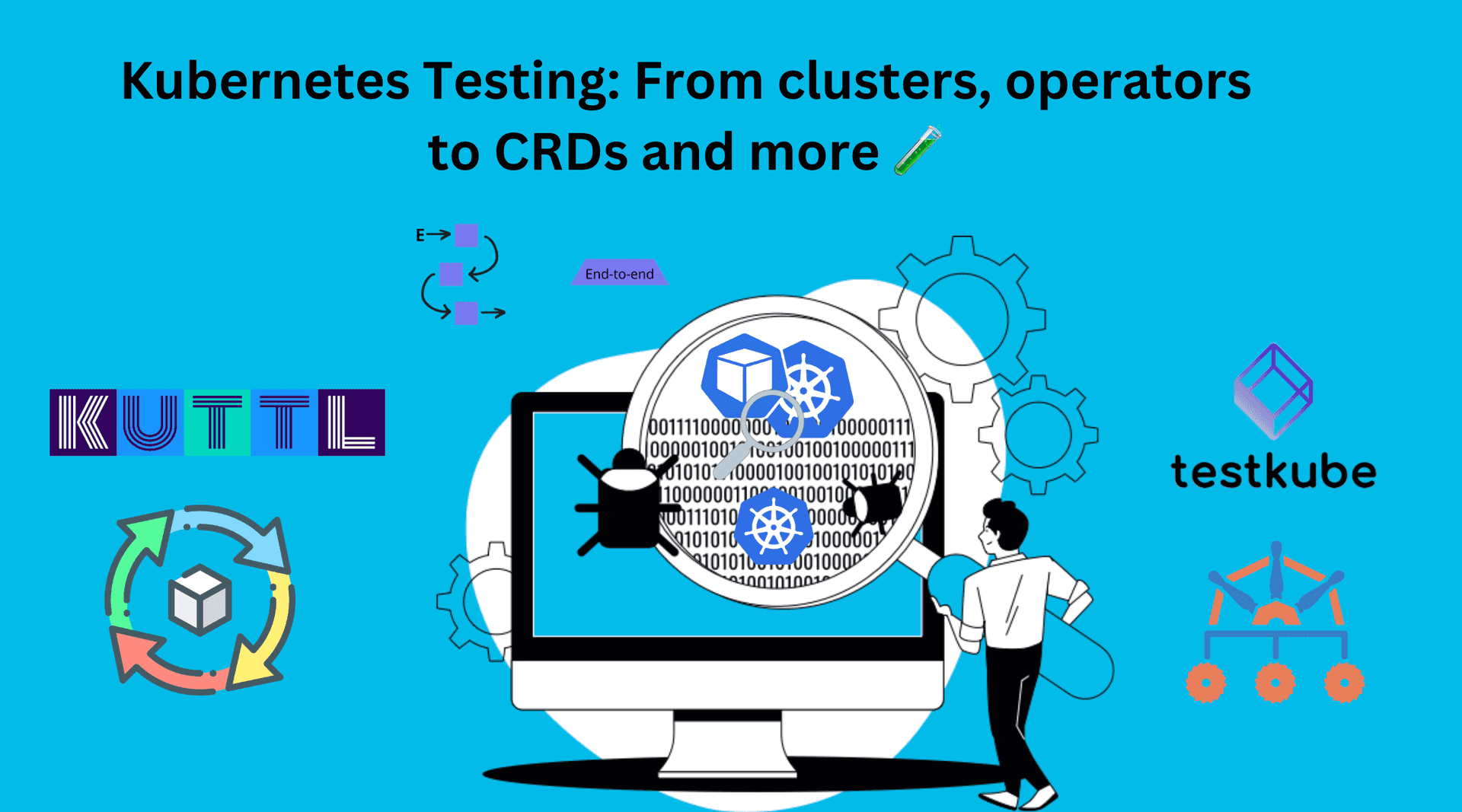
Kubernetes Testing: From clusters, operators to CRDs and more 🧪
Content
A Deep dive into tools and practices for testing Kubernetes environments
Introduction
Kubernetes is the leading platform for container orchestration, but deploying and managing a Kubernetes cluster requires thorough testing to ensure everything works correctly.
This guide will cover essential tools and practices for testing Kubernetes clusters and components, ensuring your deployments are robust and reliable.
Importance of Testing Kubernetes Clusters
-
Application Functionality: Testing ensures your applications run correctly in a Kubernetes environment. It helps identify issues with performance, functionality, and interactions with other services and databases. By testing, you can confirm that your application meets expected standards and provides a good user experience.
-
Operational Reliability: Testing evaluates the cluster's ability to handle your application under different conditions. It helps find issues like resource allocation problems, network connectivity issues, and configuration errors. Addressing these issues through testing ensures your cluster is reliable and can manage your application effectively.
-
Performance Optimization: Testing helps assess how well your application performs under various workloads and configurations. It identifies performance bottlenecks, allowing you to optimize your application to handle load efficiently.
Types of Tests for Kubernetes
-
Unit Tests: These tests focus on individual components or functions of a microservice. They are usually performed during the development phase and do not involve Kubernetes. Unit tests ensure that each part of your application works as expected in isolation.
-
Integration Tests: Integration tests check if different components of an application work together as expected. They involve testing communication between microservices, databases, and interactions with external APIs. These tests help ensure that the integrated parts of your application function correctly.
-
End-to-End (E2E) Tests: E2E tests simulate user workflows and verify that the entire application functions as expected from the user's perspective. These tests often require a Kubernetes environment to run the application components. E2E tests are crucial for validating the complete functionality of your application in a real-world scenario.
-
Performance or Load Tests: Performance tests assess the application's ability to handle load and maintain responsiveness under various conditions. These tests may involve stress testing, load testing, and benchmarking on a Kubernetes cluster. Performance tests help identify bottlenecks and ensure that your application can scale effectively.
-
Resilience Tests or Chaos Tests: These tests evaluate an application's ability to recover from failures and continue functioning. They can involve chaos testing, failover testing, and disaster recovery testing on a Kubernetes cluster. Resilience tests help ensure that your application can withstand unexpected disruptions and maintain availability.
Tools for Testing Kubernetes
KUTTL (Kubernetes Test Tool)
KUTTL is a declarative integration testing harness for testing operators, KUDO, Helm charts, and other Kubernetes applications or controllers. Test cases are written as plain Kubernetes resources and can be run against a mocked control plane, locally in kind, or any other Kubernetes cluster.
Demo:
-
Install KUTTL:
Install the kubectl kuttl plugin. To do so, please run the below commands:
brew tap kudobuilder/tap brew install kuttl-cli
Another alternative is krew, the package manager for kubectl plugins.
kubectl krew install kuttl
-
Create a Test Case:
apiVersion: kuttl.dev/v1beta1
kind: TestStep
apply:
- my-resource.yaml
assert:
- command: kubectl get myresource my-instance -o yaml
commands:
- command: helm init
unitTest: false
- Run the Test:
kubectl kuttl test
Chainsaw
Chainsaw is a tool primarily developed to run end-to-end tests in Kubernetes clusters. It tests Kubernetes operators by running a sequence of steps and asserting various conditions.
Demo:
- Install Chainsaw:
go install github.com/operator-framework/operator-sdk/cmd/operator-sdk@latest
- Create a Test Scenario:
apiVersion: chainsaw.kyverno.io/v1alpha1
kind: Test
metadata:
name: example
spec:
steps:
- try:
- apply:
resource:
apiVersion: v1
kind: ConfigMap
metadata:
name: quick-start
data:
foo: bar
- assert:
resource:
apiVersion: v1
kind: ConfigMap
metadata:
name: quick-start
data:
foo: bar
- Run the Test:
You can run the chainsaw test command:
chainsaw test
Testkube
Testkube is a Kubernetes-native testing framework that allows you to orchestrate and scale tests within Kubernetes. It supports various testing frameworks like Postman, Cypress, and k6.
Demo:
- Install Testkube:
brew install testkube
testkube init demo
- Create a Test:
testkube create test --name my-test --type postman/collection --source https://example.com/my-collection.json
- Run the Test:
kubectl testkube run test my-test
Kubernetes E2E Framework
This framework provides a set of tools and libraries to write end-to-end tests for Kubernetes. It is designed to test the functionality of Kubernetes clusters and components.
Demo:
- Clone the Repository:
git clone https://github.com/kubernetes-sigs/e2e-framework
- Write a Test:
package main
import (
"testing"
"sigs.k8s.io/e2e-framework/pkg/env"
)
func TestMain(m *testing.M) {
env.TestMain(m)
}
- Run the Test:
go test -v
Goss
Goss is a YAML-based serverspec alternative tool for validating a server's configuration. It can be used to test Kubernetes nodes and configurations.
Demo:
- Install Goss:
curl -fsSL https://goss.rocks/install | sh
- Create a Goss File:
file:
/etc/passwd:
exists: true
- Run Goss:
goss validate
...............
Total Duration: 0.021s
Count: 15, Failed: 0
Garden
Garden is a development and testing tool that allows you to define, build, and test your Kubernetes environments.
Demo:
- Install Garden:
curl -sL https://get.garden.io/install.sh | bash
- Create a Garden Project:
kind: Project
name: my-project
environments:
- name: dev
- Run Garden:
garden deploy
Conftest
Conftest is a tool for writing tests against structured configuration data using the Open Policy Agent (OPA) Rego language.
Demo:
- Install Conftest:
brew install conftest
- Write a Policy:
package main
deny[msg] {
input.kind == "Deployment"
not input.spec.template.spec.containers[_].resources
msg = "Containers must have resource limits"
}
- Run Conftest:
conftest test my-deployment.yaml
Best Practices for Kubernetes Testing
-
Automating Tests in CI/CD Pipelines: Integrate your tests into CI/CD pipelines to ensure that every change is automatically tested before it reaches production. This helps catch issues early and ensures that your codebase remains stable. Tools like Jenkins, GitLab CI, and GitHub Actions can be used to automate these tests.
-
Regularly Updating and Maintaining Test Cases: Keep your test cases up to date with the latest changes in your application and infrastructure. Regular maintenance ensures that your tests remain relevant and effective in catching new issues. Review and update your test cases periodically to adapt to any changes in your environment.
-
Monitoring and Analyzing Test Results: Use monitoring tools to keep track of your test results and analyze them for patterns or recurring issues. Tools like Prometheus, Grafana, and ELK Stack can help you visualize and analyze test data. Regular analysis helps in understanding the health of your applications and clusters, and in making informed decisions for improvements.
Conclusion
Testing is an important component in the lifecycle of Kubernetes deployments. It ensures that your clusters and applications are not only functional but also resilient and performant. By integrating comprehensive testing strategies, you can detect and resolve issues early, maintain operational reliability, and optimize performance.
Implementing robust testing practices using tools like KUTTL, Chainsaw, Testkube, and others discussed in this guide will help you achieve a stable and reliable Kubernetes environment. Regularly updating your test cases, automating tests in CI/CD pipelines, and monitoring test results are essential practices to keep your Kubernetes deployments healthy and efficient.
By adopting these best practices and leveraging the right tools, you can ensure that your Kubernetes clusters and applications are well-tested and ready to handle production workloads.
Additional resources